Adding attributes to HTML in React
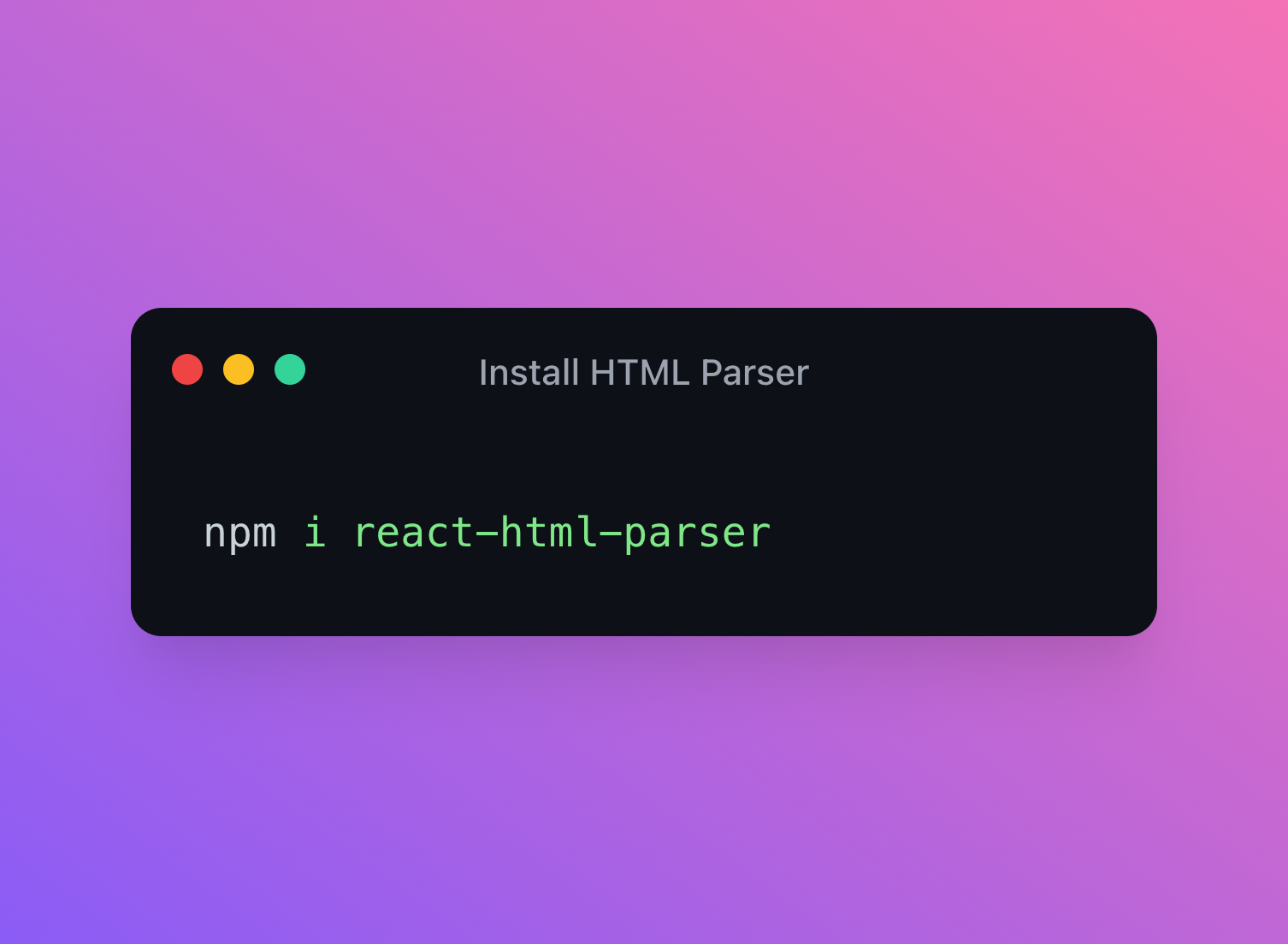
Sometimes you are served raw HTML from an API that you want to display in React and you need to modify or add attributes to certain elements inside.
For this example, we will add the target="_blank"
attribute to all anchors inside of an HTML string, which will cause the links to be opened in a new browser tab.
Parsing HTML is extremely tricky, so we will leverage an existing package for this:
1npm i react-html-parser
Once we have it installed, we'll create a new utility function to handle transforming the html, called HtmlTransformer
:
1import ReactHtmlParser from "react-html-parser" 2 3export default function HtmlTransformer(html) 4{ 5 return ReactHtmlParser(html, { 6 transform(node) { 7 if (node.type === 'tag' && node.name === 'a') { 8 node.attribs.target = "_blank" 9 }10 }11 });12}
Now we can import this into any React component if we need to transform links inside of any HTML to be opened externally:
1import HtmlTransformer from "../utilities/html_transformer"2 3export default function Article({ html })4{5 return (6 <div>{HtmlTransformer(html)}</div>7 )8}
And that's it!