Output localized dates with Blade
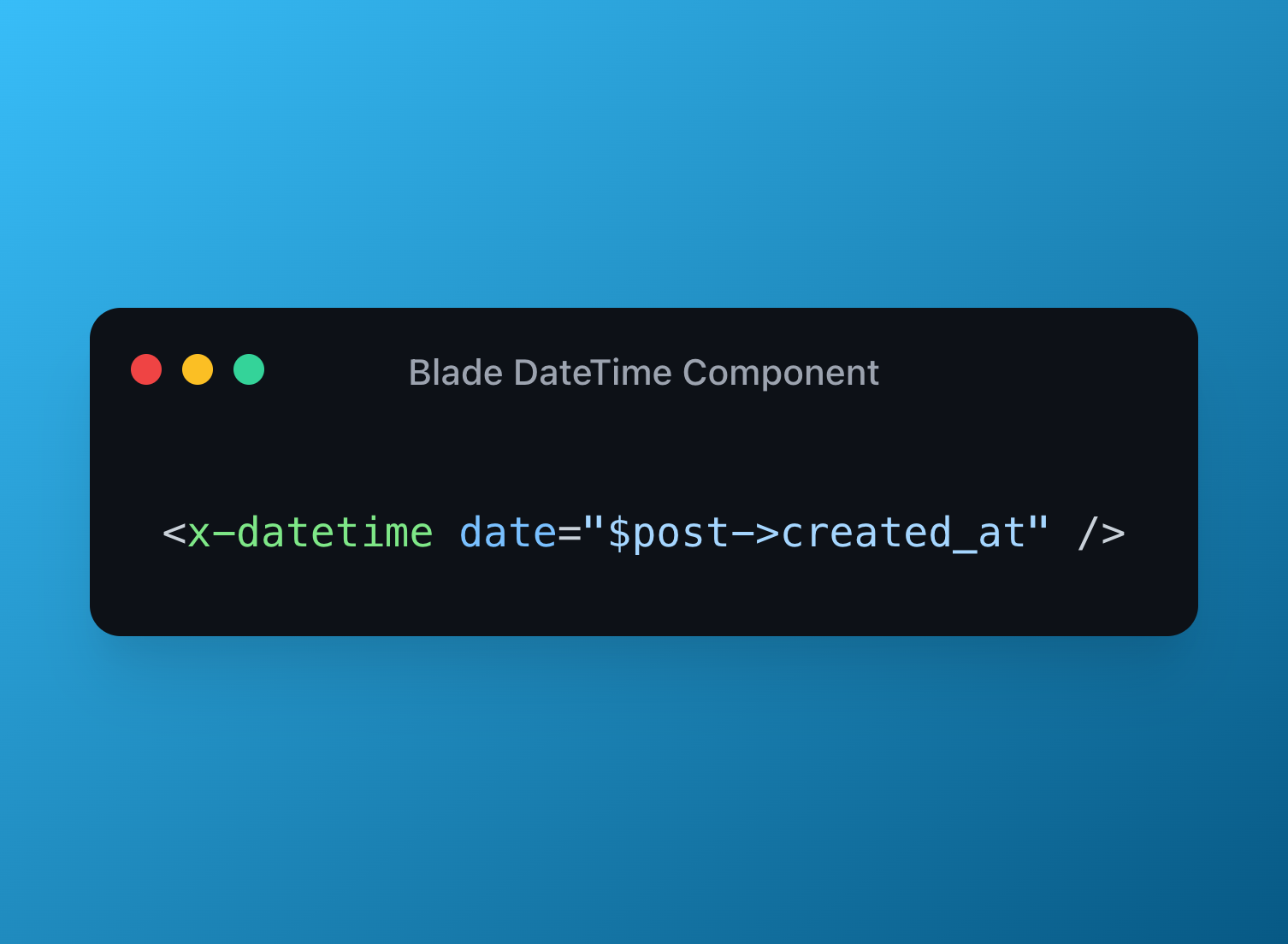
If you’re working on a Laravel app that may:
Have users logging in across the world
Be installed by developers in different timezones
Have some application dates output in a single format
Leave your applications timezone on UTC
and let a Laravel Blade component do the rest. I’ll show you what I mean.
For this, we’ll be using Laravel 7’s new Blade components feature.
Creating the Blade Component
First, let's whip up a new component. We'll call it DateTime
:
We'll generate an
inline
component using the--inline
flag. We don't need a separate view for this bad boy.
This component name allows us to render it in a really easy and memorable way in our Blade:
Now we need to be able to pass a Carbon
date into it. We'll use a parameter simply named date
.
This is what we're going for:
Looks nice, right? Let's get to the guts of the component.
The Blade Component
Laravel will generate a new class inside of your app/View/Component
directory named DateTime.php
.
We want this component to do a couple things:
Set the timezone to the users preference
Set a common date format that all dates will be output to
Copy and paste the below completed component, overwriting the generated code:
1namespace App\View\Components; 2 3use Carbon\Carbon; 4use Illuminate\View\Component; 5 6class DateTime extends Component 7{ 8 public $date; 9 10 public $format;11 12 public function __construct(Carbon $date, $format = null)13 {14 $this->date = $date->setTimezone($this->timezone());15 $this->format = $format;16 }17 18 public function render()19 {20 return $this->date->format($this->format());21 }22 23 protected function format()24 {25 return $this->format ?? 'Y-m-d H:i:s';26 }27 28 protected function timezone()29 {30 return optional(auth()->user())->timezone ?? 'America/Toronto';31 }32}
Notice in the timezone()
method, if there is an authenticated user, we will use their timezone they have saved on their user account. Otherwise, we will use a default timezone to display dates in. This would likely be a timezone you expect most of your user-base to be from.
The above implementation assumes you have created a
timezone
column on yourusers
table that will be available for users to set. This gives a lot of flexibility. For example, if someone who travels often is using your app, they could simply change their timezone and see all outputted application dates in their time, rather than the servers time.
Now we can stop writing this to output dates in all of our views:
1{{ $post->created_at->format("Y-m-d H:i:s") }}
And write this instead:
1<x-datetime date="$post->created_at"/>
We can also set the format
parameter if we needed to override the default format:
1<x-datetime date="$post->created_at" format="Y-m-d"/>
Blade components have such great possibilities for cleaning up these types of operations in our view files. I'm seeing more and more opportunities as I continue to work with Laravel 7.
Hope you enjoyed this one!